Reverse String
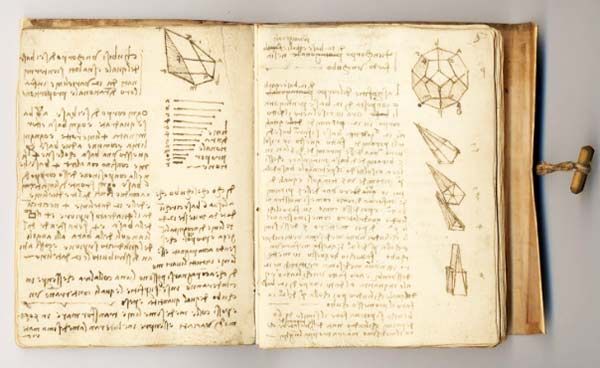
Reversing a string is another classing programming problem. Let's assume you get as input the string "algorithm", the goal is to write code that will return "mhtirogla".
Before implementing
Depending on the language, there are few questions you might need to ask yourself before you start:
1. Should you do the reversing in place?
If you are using a language where strings are mutable that is almost always the case or it would be just too easy :)
C, C++, Objective C, PHP and Ruby are languages where strings are mutable, meaning you can change the value you're holding in the variable.
As mentioned in our previous problem, languages like Go, C#, Java, Javascript have immutable strings meaning that you cannot change the value itself and will be generating new values whenever you change the contents of a string. For these languages you won't be able to reserve the string in place, as it's not possible.
2. How are strings implemented in the language?
In C there is no such a thing as string and normally strings are implemented as an array char[]
or pointer char *
. Because of they way arrays work in C, you can't really tell when you've got to the end of it. So it was defined that strings in C are an array of 'char' ended by a null
(\0) character. C++ has a string class but it is implemented as a null terminated array of chars. That means that when implementing this same solution in C/C++, you need to be careful to reverse all characters but to keep the null character at the end of the string.
3. What is the character range we have to support?
This question will come up almost every time we discuss a string problem.
Go, Python >= 3 and Ruby >= 1.9 support unicode (multi-byte characters) while C and C++ don't (in case the of C++ this is a bit trickier), so you will need to extra careful in those languages in case you are implementing your solution in them.
The Solution
We've discussed some very deep things above, however the actual solution for this problem is pretty trivial:
func reverseString(str string) string {
strLen := len(str)
strByte := make([]rune, strLen)
for idx, r := range str {
strByte[strLen-1-idx] = byte(r)
}
return string(strByte)
}
I'm creating a byte array to hold the runes of the string and then looping through the actual string and adding them in reverse order to the byte array. When we're are done, and return the byte array, not forgetting to cast it to a string before returning.
You can find the complete solution at our repository.